Where Actually Are The Antipodes?
metadata
- keywords:
- published:
- updated:
- Atom Feed
Recently I was thinking about where actually is the antipode of the UK?
and where actually is the antipode of the US?
. I decided to write a little script to overlay the world on top of itself so that it would be easy to find each location’s antipode. I know that the Wikipedia article on the antipodes has such an image but I dislike it aesthetically as it only shows half the world (I realise only half the world needs to be shown but I just find it un-intuitive). This one is far better but of low quality, in my opinion.
The Python script that I wrote to achieve this is shown below. It is quite a bit longer than what it ideally should be because I ran into problems with countries that cross the meridian (the UK, France, Spain, Algeria, Mali, Burkina Faso, Togo, Ghana and Antarctica) not being plotted when they are transformed to their antipode. This is an example of software problems with the anti-meridian and needed to be fixed. My chosen way was to: split the world up into quadrants (positive and negative longitude and latitude); determine if a shape was going to pose a problem when transformed; find the four overlaps of the shape with the four quadrants; and plot them individually. This way no single shape would cross the anti-meridian (or the equator).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 |
|
checkout
the “main” branch).The image produced by this script is shown below.
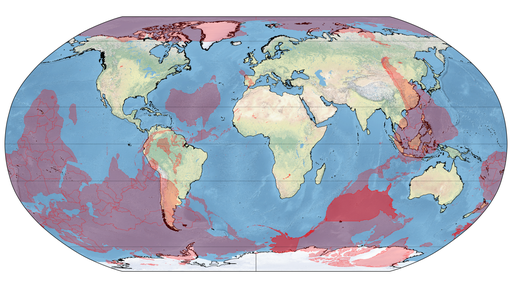
A couple of things strike me when I look at this image:
- Australia is a lot closer to the equator than I thought. As a child I always thought that I’d end up in Australia if I kept on digging - this is simply not the case. The transform of the UK is off the south-east coast of New Zealand by a long way.
- The transform of Tasmania is almost exactly in-between the UK and the US.
- The Pacific Ocean is huge. Brazil, Africa, the Middle East, Europe, the Indian sub-continent, central Asia and half of Russia are all within the antipode of the Pacific Ocean. I think the immense size of the Pacific Ocean is lost on most map projections that put the meridian in the centre.