How To Avoid Places
metadata
- keywords:
- published:
- updated:
- Atom Feed
The eagle-eyed amongst you may have noticed something interesting in the previous blog post: I chose to highlight some countries in particular (the UK and the US) and I chose to call this list avoids
in the source code (line 19).
Whilst the previous script did indeed easily display where the antipode of any point was (thus helping me to find that Tasmania would be a great bet) it did have one huge flaw if it was to be used to avoid places: it would only tell you how to avoid a point. The script was not helpful at all at telling you how to avoid an area, such as a country: I therefore set out to write version 2 of my script.
My chosen method was to expand the coastlines of a list of countries by set distances. By successively increasing the coastlines I would create an area on the far side of the Earth that was further away than the expanded coastline (or indeed outside this “exclusion zone”). I could then increase the distance until there was nowhere left on the surface of the Earth that was that far away from the coastlines. The technical term for such an operation is to buffer an object and Shapely has a function to do just that.
Unfortunately, the Shapely function has no knowledge that it is being used to describe areas on the surface of the Earth: it is just a library that describes shapes. Consequently, the buffer function expands objects by whatever unit the object is described in, in this case: degrees. This poses a major problem: the length of a degree changes depending on where you are in the world and which direction you would like to go. Additionally, it has no knowledge of the anti-meridian and the problem that it poses. What I needed was a function that took in an area defined in degrees and buffered it by a single distance in kilometres: the resultant object would be buffered by a varying amount in units of degrees but by a constant amount in units of kilometres. For this script to work I wrote two functions:
pyguymer3.geo.bufferSrc.buffer_Point()
: uses Vincenty’s (direct) formula (as found inpyguymer3.geo.calc_loc_from_loc_and_bearing_and_dist()
) to buffer a single point by going a set distance away from the point for a range of angles across the whole compass. By connecting these points together an ellipse-like area is found around a single point.pyguymer3.geo.bufferSrc.buffer_Polygon()
: usespyguymer3.geo.bufferSrc.buffer_Point()
to buffer each point in each (external and internal) edge of a polygon and then finds the union of all of the ellipse-like areas (and the original polygon) so as to find the buffered polygon.
Once these two functions were written (and extra logic was added to deal with areas overlapping the anti-meridian) it was relatively easy to buffer the coastlines of the UK and the US and find out where in the world to go if you wanted to avoid them. Below is the script that I wrote that uses these two functions (I found that simplifying the polygons whenever I could drastically reduced runtime).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 |
|
checkout
the “main” branch).The image produced by this script is shown below.
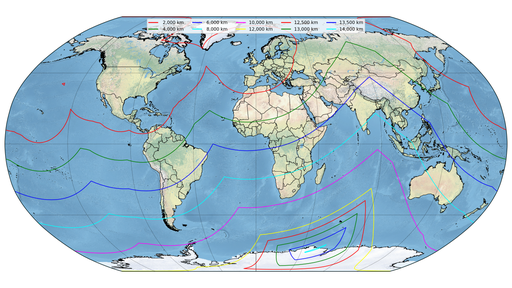
A couple of things strike me when I look at this image:
- You can only get roughly 14,000 km away from both the UK and the US even though the half-circumference of Earth is roughly 20,000 km.
- The Kerguelen Islands are your best bet (you’ll have to ask the French very nicely if you want to visit).
- Alaska looses significance the further away you get (to be expected) but somewhere between 10,000 km and 12,000 km it becomes irrelevant. Note the straight green (4,000 km) line that cuts across South Korea - that is the buffered Alaskan coastline. Similarly, the straight blue (6,000 km) line that cuts across China is the buffered Alaskan coastline too. You can follow that straight line as it steps through Asia, however, it becomes smaller and smaller as the coastlines from the south east of England and Hawaii expand - the buffered Alaskan coastline finally becomes encompassed by the other coastlines somewhere in the Indian Ocean.
- Whilst the previous blog post showed Tasmania as being between the UK and the US it would, in fact, be a bad choice because it is far too close to Hawaii on the other side (it only survives roughly 8,500 km).